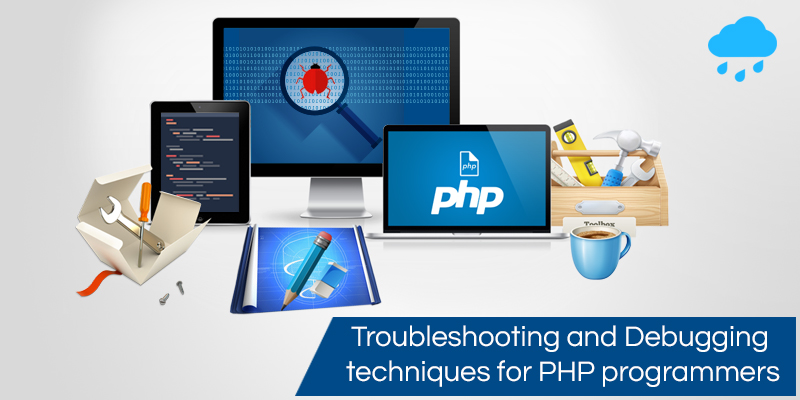
First thing to do while troubleshooting your code is enabling error reporting.
You can start by including the below two lines of code at the start of your PHP script.
ini_set('display_errors',1);
error_reporting(E_ALL);
There are four types of errors in PHP.
1. Syntax Errors
Syntax errors are caused by typo in your code. A missing semicolon, parenthesis or quotation mark may result in syntax error.
2. Fatal Errors
Most common fatal error occurs while coding are of undefined function or class. The error itself tells you which function or class is not defined.
3. Warnings
Warnings often appears when you include a non existing file or pass incorrect number of parameters to functions etc.
4. Notices
A common notice you will see while coding is of “Undefined index”. These notices are helpful while debugging the code.
Things to remember while coding
1) Semicolons.
Leaving out the semicolons will end up with “Parse error” while running your PHP script.
The semicolons completes a PHP statement. PHP reads a statement until the semicolon or the PHP closing tag. PHP doesn’t care how many lines of code you have written or how many blank spaces you have in your code. PHP checks the code until the next semicolon and treats everything before the semicolon as a single statement.
Even though semicolons are not needed for the last PHP statement before the closing tag, It is always a good practice to put the semicolons at the end of each statements.
2) Dollar sign.
A variable must start with a dollar($) sign. Make your script easier to understand by using descriptive variable names like $firstName, $address, $dateOfBirth instead of $var1, $var2, $var3. This will help you in troubleshooting the script. This will also make your script understandable by a third person.
3) Single/Double Quotes.
Everything inside the single quotes are treated as plain string while things inside double quotes will parse the variables by replacing them with their values.
$firstName = "Sam";
echo 'Hello $firstName'; // Output: Hello $firstName
echo "Hello $firstName"; // Output: Hello Sam
4) Keywords.
Constants should not be given names that are PHP Keywords. Keywords have certain meaning and PHP treats them as PHP feature of the same name. Some of the PHP keyword are echo, print, continue, function etc.
Here is the list of complete PHP keywords
There are reserved constants as well. Here is the full list of reserved constants
Debugging techniques
1) echo $var; – use echo to print the variable so that you can confirm the value in that variable. You can use this in POST/GET handling to confirm whether the script has expected value in POST/GET.
2) var_dump ($var); – var_dump is useful while dealing with arrays and objects. It displays their complete structure so that you can check if the array or object has the correct value.
3) die (“value in var: $var”); – die will end the script after printing the message. You can use this anywhere in your script while debugging.
Always remember to remove your debugging code once you are done with it.
Leave a Reply
You must be logged in to post a comment.