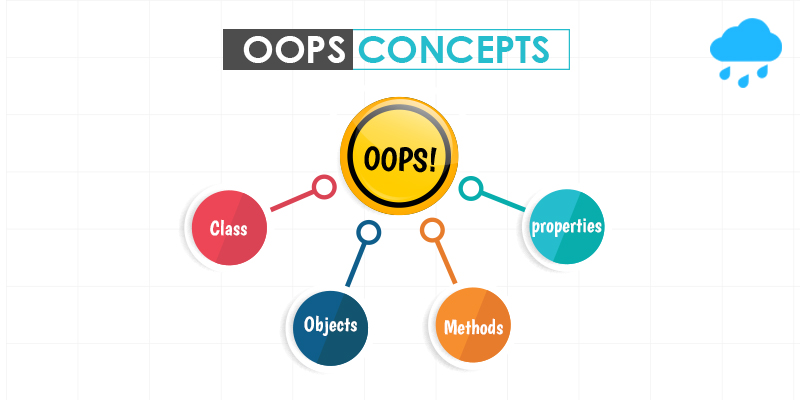
Object-oriented programming (OOP) is more advanced and efficient way of programming than the procedural style. OOP helps you in better code organization and reduces repeat of code. OOP concept was added to php5, to build complex and reusable web applications.
The OOP in PHP starts by creating a class. Here is how we create a class.
1) Create a class
class Vehicle {
// PHP codes
}
2) Adding properties to the class
class Vehicle {
public $color = “Black”;
public $type = “Hatchback”;
}
3) Create objects from a class
We create a new object by using the keyword “new”.
$fiat = new Vehicle();
We can create more than 1 object of the same class. Each of them with their own set of properties.
$fiat = new Vehicle();
$honda = new Vehicle();
Here we have created two new objects “fiat” and “honda” from the same class “Vehicle”. All the objects created from the class will have same methods and properties with different values assigned to them.
4) Get an object’s properties
We can get an object’s properties like below:
echo $fiat -> color;
echo $honda -> type;
Output:
Black
Hatchback
5) Adding methods to a class
A function inside a class is called Methods.
class Vehicle {
public $color = “Black”;
public $type = “Hatchback”;
public function helloworld()
{
return “Hello”;
}
}
Here is how we call a method.
echo $fiat -> helloworld();
echo $honda -> helloworld();
Output:
Hello
Hello
Leave a Reply
You must be logged in to post a comment.